Unicode in java is known as the 16-bit character programming standard and can easily represent each and all characters in the world of recognized languages. Unicode bold resolution to create a small set of characters, incorporating every discerning writing method from around the world. However, Unicode is not a simple 16-bit character programming. It has different ways of thinking in terms of characters, and you have to know the Unicode way of thinking about things.
The essential aim of Unicode is to amalgamate dissimilar linguistic indoctrination arrangements so that it can stop misperception amongst processer methods. That use limited encoding principles some of them are EBCDIC, ASCII, and so on. The development of Java Unicode is when the Unicode principles are known for its quite lesser set of characters. Java an extensively used coding language specifically made for use in the dispersed atmosphere in the world of the internet.
Let’s know why Java uses Unicode—
A computer structure can be empowered to store numbers and text that can be understood by humans, however, there must be a code that replaces numbers with letters. Unicode defines as generic and defines the appropriate code using character programming. Character programming is the process of converting characters into a number that is also for each character.
The main purpose of Unicode is always to integrate different language programming patterns. So that it does not create any confusion in computer systems using fewer programming methods like EBCDIC, ASCII, etc.
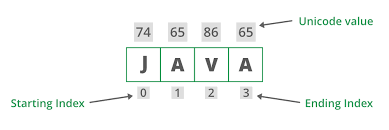
Before the advent of Java Unicode there were numerous language standards
ASCII (American Standard Code for Information Interchange
ISO 8859-1- these were used by the Western European Language.
KOI-8 –this was for Russian.
GB18030 and BIG-5- this was for Chinese, etc.
ASCII In JAVA
ASCII (American Standard Code for Information Interchange) is a character encoding system used to represent text in computers and other devices. It assigns a unique numerical value to each character, including letters, numbers, punctuation marks, and other symbols. In the ASCII system, each character is represented by a 7-bit binary code.
It can only represent a maximum of 128 characters because it is a seven-bit code**. There are 95 printable characters now defined by it, including 26 upper cases (A to Z), 26 lower cases, 10 numbers (0 to 9), and 33 special characters such as mathematical symbols, punctuation marks, and space characters.
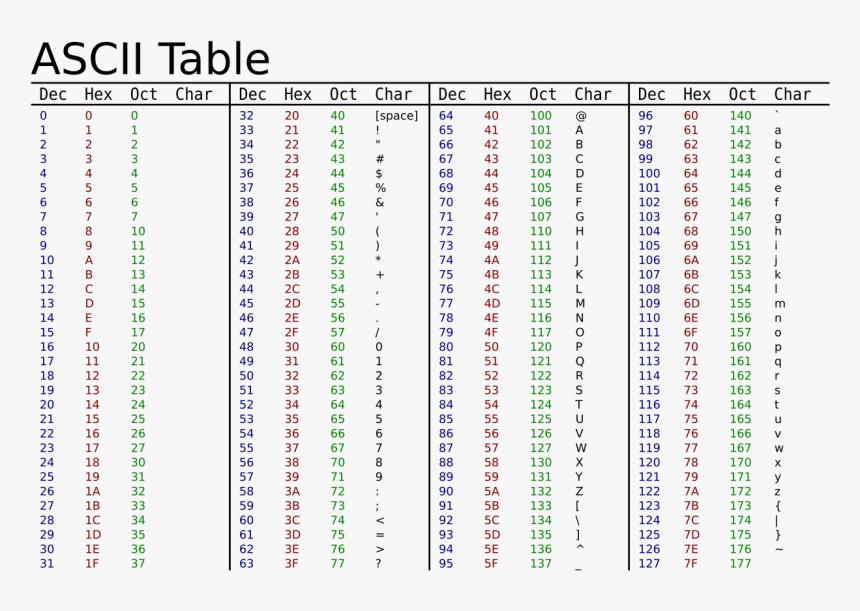
How to Print ASCII in Java?
ASCII (American Standard Code for Information Interchange) is a system that assigns unique numerical values to characters so that computers can store and manipulate them. The assigned numerical values are represented in binary form for use by electrical equipment, since it is not possible to use or store the original character form.
There are four methods available in Java to print the ASCII value. Each method is briefly explained below, along with an example of how to implement it:
- Brute force technique
- Type-casting method
- Format specifier method
- Byte class method [Most Optimal]
Method 1 – Using Brute Force Method
Simply assign the character to a new integer-type variable to determine the character’s ASCII value. Java automatically inserts the character’s ASCII value into the new int variable.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// Create an instance of the scanner class
Scanner sc = new Scanner(System.in);
// get character input from the user
char c = sc.next().charAt(0);
// assign c to an int variable
int ascii = c;
System.out.println("ASCII value of " + c + ": " + ascii);
}
}
JavaScriptOutput —
b
ASCII value of b: 98
JavaScriptMethod 2 – Using the Type-casting Method
In Java, type-casting is a technique for changing a variable’s datatype. To use type casting to get the ASCII value of a character in Java, you can follow these steps:
- Declare a character variable and assign a value to it.
- Type cast the character variable to an integer using (int).
- Print the ASCII value using System.out.println().
public class Main {
// Main driver method
public static void main(String[] args) {
// Character whose ASCII value is to be computed
char ch = '}';
// Typecasting the character to int and
// printing the same
int asciiValue = (int) ch;
System.out.println("The ASCII value of " + ch + " is: " + asciiValue);
}
}
JavaScriptOutput —
The ASCII value of } is: 125
JavaScriptMethod 3 – Using the format() Method
The format() method in Java is used to format a string using a specified format string and arguments.
The sprintf() function in the c programming language and the format() method in python achieve similar functionality.
In this method, a format specifier is used to produce the ASCII value of the given character. By designating the character to be an int, we were able to save the value of the supplied character inside a format specifier. As a result, the format specifier contains the character’s ASCII value.
// Importing format library
import java.util.Formatter;
public class Main {
public static void main(String[] args) {
// Character whose ASCII value we want to compute
char character = 'a';
// Initializing the format specifier
Formatter formatSpecifier = new Formatter();
// Converting the character to an integer and
// ASCII value is stored in the format specifier
formatSpecifier.format("%d", (int) character);
// Print the corresponding ASCII value
System.out.println(
"The ASCII value of the character ' " +
character +
" ' is " +
formatSpecifier
);
}
}
JavaScriptOutput —
The ASCII value of the character ' a ' is 97
JavaScriptMethod 4 – Using getBytes() Method
This is the most optimal way to get the ASCII value of a character
- Initialize the character as a string.
- Create an array of type bytes using getBytes() method.
- Print the element at the 0th index of the byte array.
This technique is typically used to translate an entire string to its ASCII values. The try-catch is provided for characters that violate the encoding exception
// Importing I/O library
import java.io.UnsupportedEncodingException;
public class Main {
public static void main(String[] args) {
// Try blocking to check the exception
try {
// Character is initiated as a string
String sp = "a";
// An array of byte type is created// by using getBytes method
byte[] bytes = sp.getBytes("US-ASCII");
/*This is the ASCII value of the character
/ present at the '0'th index of the above string.*/// Printing the element at '0'th index// of array(bytes) using charAt() method
System.out.println(
"The ASCII value of " + sp.charAt(0) + " is " + bytes[0]
);
}// Catch block to handle the exception
catch (UnsupportedEncodingException e) {
// Message printed for exception
System.out.println("UnsupportedEncodingException occurs.");
}
}
}
JavaScriptOutput
The ASCII value of a is 97
JavaScriptConclusion
The integration of Unicode and ASCII support in Java underscores the language’s commitment to flexibility, accessibility, and global compatibility. Whether developers are creating applications for English-speaking users or catering to multilingual audiences worldwide, Java’s robust character handling capabilities empower them to build software solutions that can effectively communicate and interact across linguistic and cultural boundaries. As technology continues to evolve and globalization becomes increasingly prevalent, Java’s Unicode and ASCII support remain essential pillars of its versatility and adaptability in the ever-expanding digital landscape.
Frequently Asked Questions
Ans: Unicode in Java refers to the standard character encoding scheme used to represent text. It allows Java applications to handle a wide range of characters from different languages and symbol sets.
Q2. How does Java handle Unicode characters?
Ans: Java internally represents strings using Unicode encoding, typically UTF-16. This allows Java programs to work seamlessly with characters from various languages and scripts.
Q3. What are ASCII values in Java?
Ans: ASCII values in Java refer to the numeric representations of characters based on the ASCII (American Standard Code for Information Interchange) encoding scheme. ASCII values range from 0 to 127 and represent characters such as letters, digits, and symbols commonly used in the English language.
Q4. How can I find the ASCII value of a character in Java?
Ans: You can find the ASCII value of a character in Java by casting the character to an integer. Since Java’s char type is based on Unicode, characters within the ASCII range will have ASCII values identical to their Unicode code points.
Q5. Can Java handle characters from languages other than English?
Ans: Yes, Java’s Unicode support enables it to handle characters from virtually all writing systems, including languages other than English. This allows Java applications to support internationalization and localization efforts.
Q6. What is the difference between Unicode and ASCII in Java?
Ans: The main difference between Unicode and ASCII in Java lies in their encoding schemes and character ranges. Unicode supports a much wider range of characters from various languages and symbol sets, while ASCII is limited to characters primarily used in the English language.
Q7. How do I represent Unicode characters in Java source code?
Ans: Unicode characters can be represented in Java source code using escape sequences of the form uXXXX, where XXXX is the hexadecimal code point of the Unicode character.
Q8. Can I use Unicode characters in Java identifiers?
Ans: Yes, Java allows Unicode characters to be used in identifiers, such as variable names, method names, and class names. This can be useful for naming elements in code with meaningful names from different languages.